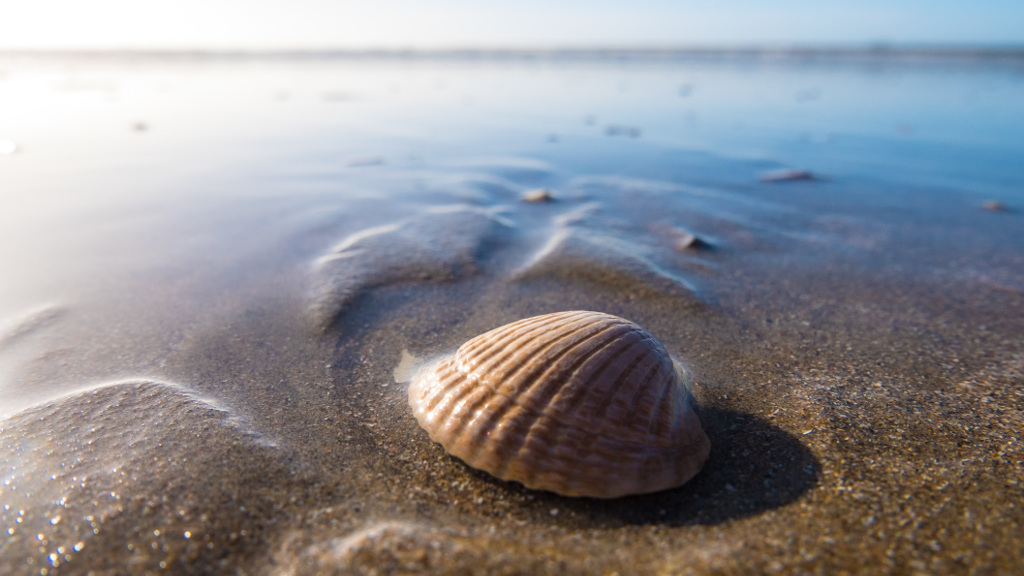
You are reading this because you can’t understand why os.getenv('SOMEVAR')
doesn’t work when echo $SOMEVAR
does; or because you’ve never figured out why this mysterious shell command, export
, is needed.
The shell is the software that gives you a prompt and parses and executes the commands you type. But the shell is also a programming language, and, like Python, it has variables. So, here is a version of hello world in Python:
import sys greeting = "Hello, world!" sys.stdout.write(greeting) |
Here is how we would write that program in the shell (there are many different shells, but here we assume bash, which is the most commonly used in GNU/Linux systems):
greeting="Hello, world!" echo $greeting |
Variables such as greeting
are called shell variables and they have nothing to do with the environment. In the following example, Python isn’t going to pass the greeting
variable to myprogram
:
import subprocess greeting = "Hello, world!" subprocess.call('myprogram') |
Likewise, the shell isn’t going to pass the greeting
variable to myprogram
in this:
greeting="Hello, world!" myprogram |
If you want greeting
to become an environment variable, you need to “export it to the environment”:
export greeting |
The above works when greeting
is already defined. Usually it is convenient to define it and export it at the same time:
export greeting="Hello, world!" |
Environment variables usually also work as shell variables. In addition, if greeting
is already exported, then executing greeting="Good morning!"
will change the environment variable! Finally,
unset greeting |
will delete both the shell variable and the environment variable.
Another way to set an environment variable is like this:
greeting="Hello, world!" myprogram |
With this syntax, the greeting
environment variable is set and is passed (along with any other environment variables) to myprogram
, and then it is deleted immediately.
We usually use all caps for environment variables. You can use the shell env
command to view all the environment variables.